The essence of objects
CS 3500, Fall 2016
The Object Model
Every system is made of smaller sub-systems or components
System working = components working with each other
A component
Is a logical grouping of objects
Has a well-defined purpose and functionality
Completes a task by using its own functionality, or delegating to other components
An object
Is the basic “functional unit” in the object model
Has a singular, well-defined purpose
Communicates with other objects by passing messages (i.e. calling methods)
The Object Model outside software
We think of the computer as “one unit”
Set of components working together
A well-defined purpose
RAM: temp memory
Disk drive: perm memory
Processor: process
A well-defined boundary
RAM doesn’t process
Disk doesn’t cool system
The Object Model outside software
Hospital has various departments
Each department has well-defined purpose
Orthopedics
Cardiac Unit
Each department has a well-defined boundary
Cardiac unit doesn’t treat fractures
To a patient, the hospital is one unit
How are objects defined?
Each object has a specific purpose
Maintain customer records
Validate a credit card
“Behave like a stack”
Each object is created so that it can be used by other objects in a specific way
Object A assumes object B can handle a particular task
Objects of the same “type” are from the same class
Class=data type, Object=actual thing
The object model
Each object has
State
Behavior
State of an object
Each object has some data associated with it
Members or variables of the object
Values of that data together decide the “state” of the object
Behavior of the object
Each object has capabilities to operate on its or other data
Operations are in the form of methods or functions
“Behavior” changes “state”
The object model
Creating an object
Declare a variable of the class type
Create an object from that variable
Often the two steps are in one line...in C++, not in Java
Every object gets its own copy of variables and methods
Except static objects
Type of an object
The class of which this object is (inheritance makes this somewhat complicated)
Object-oriented Methodology:
Abstraction
Objects expose only those details that are necessary for other objects to know
Make themselves appear simpler than they really are
Why?
To adhere tightly to the expectations from an object
If A assumes B can perform a task, B exposes just enough functionality for A to use, no more
To hide its own complexity
Interface of an object
Its “public” face
“Interface abstracts implementation”
Object-oriented Methodology:
Abstraction
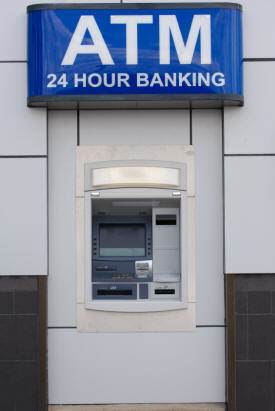
Object-oriented Methodology:
Abstraction
Object-oriented Methodology:
Abstraction in Design
Design the interface for each object first
Design one object in detail using only interfaces of the others
Details of only one object, interfaces of others
Separate the “what” from the “how”
“What”: what can an object do? (the interface)
“How”: how does the object do it? (details)
“What” is used in design: Just care about what other objects do, not how they do it
Object-oriented Methodology:
Standardizing an Abstraction
Standardize method names, parameters and output
Java: abstract class and interfaces
Abstract class: One or more methods are abstract
abstract class X
abstract public void methodname(…);
Interfaces: merely the method signatures
interface X
Object-oriented Methodology
Abstraction and Hierarchy
Creating a hierarchy...
System
Sub-systems
Components
Objects
Each level of the hierarchy exposes more details than the one above
Provides for the proper level of understanding
Object-oriented Analysis and Design by Grady Booch
Object-oriented Methodology
Encapsulation
Combining everything necessary for a cohesive entity (data+operations) into a class
Hiding all the (bitter) details within a capsule
Classes that represent self-complete entities = good encapsulation
Object-oriented Methodology
Information Hiding
Hiding all the details that are not necessary to use the object
Other objects
Good information hiding: expose only what others can use
Information hiding creates the abstraction
Information hiding in Java
Access modifiers:
private
,protected
,public
, defaultRule of thumb: make all variables
private
, only some methodspublic
Accessors and modifiers
Interface
The set of methods and variables of an object that another object can see and use
Object-oriented Methodology
Cohesion
Objects that represent an entity should contain all the functionality within it for that entity (and nothing else)
Good encapsulation
Bad/Low cohesion: classes that don't completely represent one entity, classes with multiple purposes
Classes with no self-sufficient role
E.g. classes with methods that change external data only
Classes with multiple purposes/roles
E.g. class that mixes operations and I/O
Object-oriented Methodology
Decoupling
Objects should use each other only when needed
Bad/Low decoupling: State of one object being manipulated directly within several objects
Effects of Low cohesion and decoupling
Compromises functional separation of various objects
Code and structure cluttered, complicates testing, maintenance
Characteristics of a Well-Designed Object-Oriented System
Objects/components with clear, singular purpose
Objects/components with well-defined interactions
Good encapsulation
Effective use of abstraction through information hiding
High cohesion, high decoupling
How does all of this improve our lives?
Advantages of the Object Model
Division of the system into well-defined components
Well-defined interaction within the system
Easier code reuse
Easier system integration
Applicable to all types of sub-systems
Advantages of the Object Model:
Division into components
Semantic division into components (objects)
Division into procedures is more awkward
Good if cohesion/decoupling is high
Independent development and testing
Each object is self-sufficient and purposeful
Each component can be independently stabilized and made robust
If object boundaries are well-defined
Resilient to later changes
Future upgrades change only some classes
Changes are modular and isolated
If cohesion/decoupling is high
Advantages of the Object Model:
Well-defined interactions
Objects interact with each other by message passing
Calling each other methods
Method names, signatures decided during design
Enforcing this in "structured" design is difficult
Good design disciplines interactions
Well-defined message passing
Effect of change is deterministic and finite
If decoupling is high
Interaction and cohesion: a balance
Advantages of the Object Model:
Code re-use
Using readymade code is always preferable
Less time and effort than re-inventing the wheel
Less testing, higher reliability
Provided code is from a reliable source
The basis for all readymade packages and libraries
Object re-use more convenient than procedural re-use
Objects well-defined components
Pick-and-choose re-use possible because of overriding
Well-defined interface, more amenable for reuse
Advantages of the Object Model:
Easier System Integration
Early dependency detection
During analysis and design
Concurrency and version control easier
Versions of objects instead of files
Integration testing is separable and delayed
Using “placeholders” is easier to delay integration testing
Known message passing interfaces from design
Integration process is smoother
Well-fitting, “pre-conceptualized” parts
Advantages of the Object Model:
Applicable to all types of subsystems
User interface design
Data access design
Processing workhorses