The java.lang.Appendable
interface
For objects to which characters can be appended or written:
/**
* An object to which {@code char} sequences and values can be appended.
*/
public interface Appendable {
/**
* Appends the specified character to this {@link Appendable}.
*/
Appendable append(char c);
/**
* Appends the specified character sequence to this
* {@link Appendable}.
*/
default Appendable append(CharSequence cst) {
...
}
}
Examples: PrintStream
, FileWriter
, LogStream
, ...
Generifying output code
Works only with PrintStream
s:
void dumpHashtableStats(PrintStream out) {
out.print(getHashtableStats().toString());
}
Works with any Appendable
(including PrintStream
s):
void dumpHashtableStats(Appendable out) {
out.append(getHashtableStats().toString());
}
StringAccumulator acc = new DoublingStreamAccumulator();
ht.dumpHashtableStats(acc); // Doesn't work!
Recall our StringAccumulator
interface
public interface StringAccumulator {
void add(char c);
String stringValue();
}
Suppose we can't change dumpHashTableStats
and StringAccumulator
.
Can we still use a StringAccumulator
with dumpHashtableStats
?
An adapter!
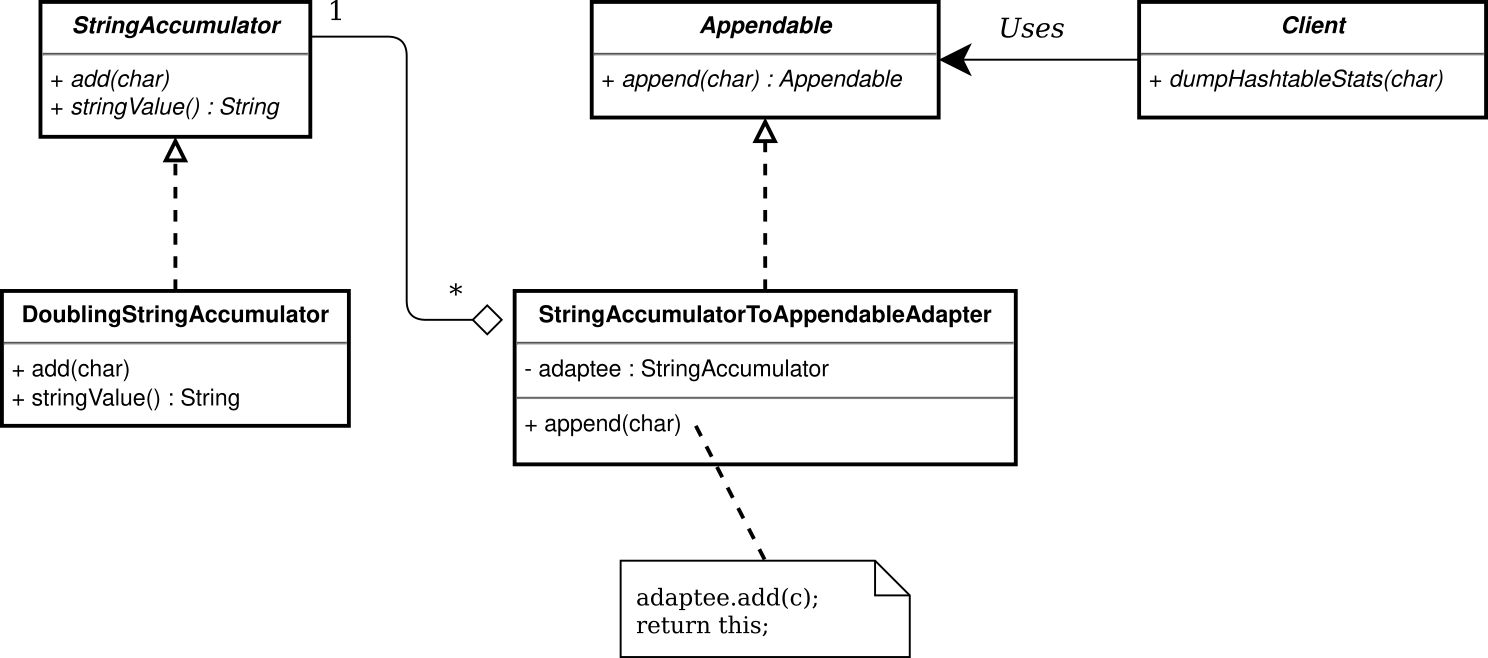
A StringAccumulator
to Appendable
adapter
public final class StringAccumulatorToAppendableAdapter
implements Appendable
{
private final StringAccumulator adaptee;
public
StringAccumulatorToAppendableAdapter(StringAccumulator acc)
{
Objects.requireNonNull(acc);
adaptee = acc;
}
public Appendable append(char c) {
adapter.add(c);
return this;
}
}
The object adapter pattern
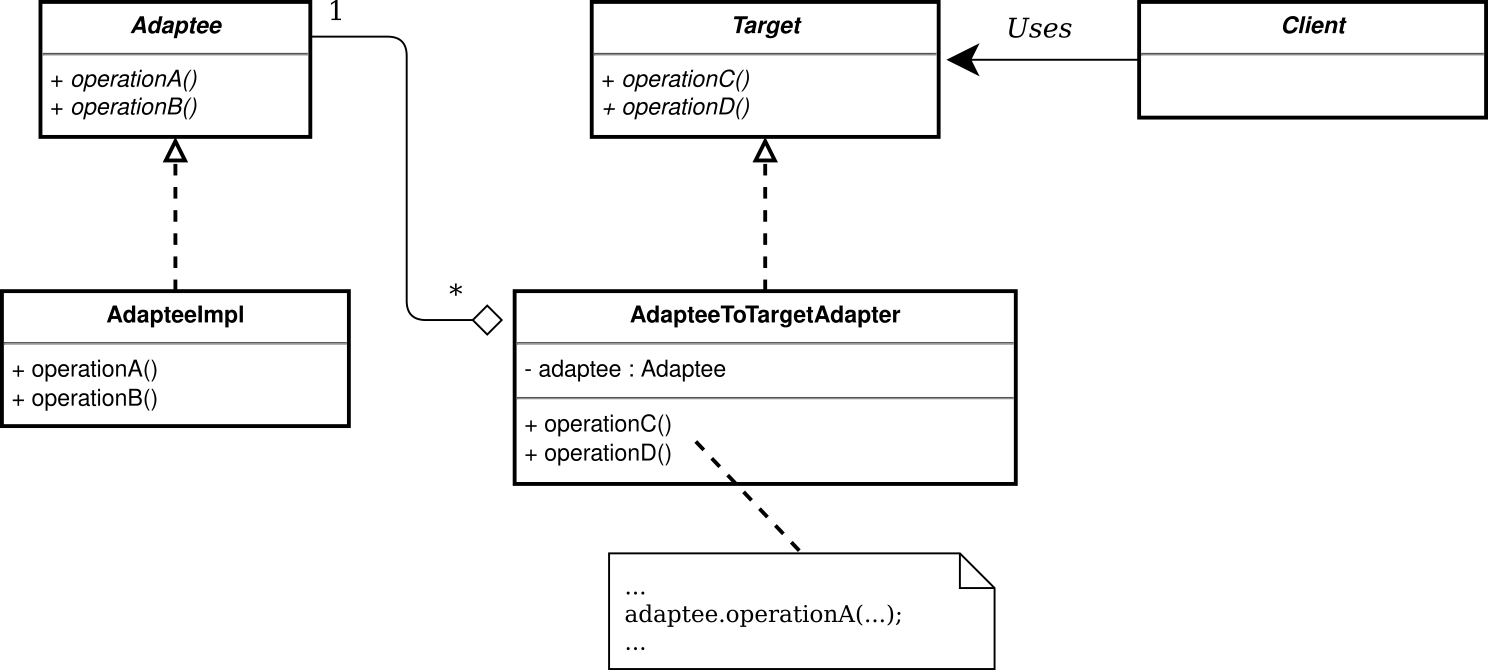
The class adapter pattern
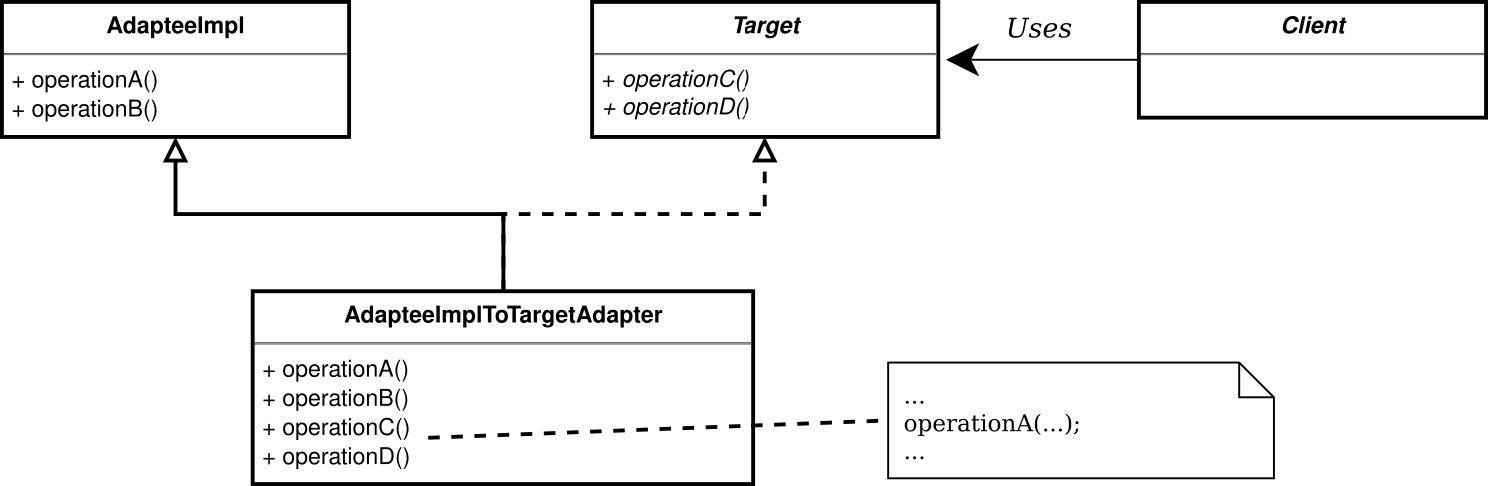